Quick start
Ready to dive into the Sprinto Developer API without drowning in details? You're in the right place.
Things to know before
While our API documentation is beginner-friendly, let's establish a baseline. We'll throw in links to relevant documentation whenever we introduce a new concept.
To get the most out of our documentation, it's good to have a basic understanding of GraphQL. If you're new or need a refresher, head to the GraphQL learning resource to brush up on your GraphQL skills.
1. Generate an API key
Any access to the Developer API is authenticated with a valid API key to ensure only authorized stakeholders get access.
Sprinto users with admin privileges can generate an API key from the Sprinto app.
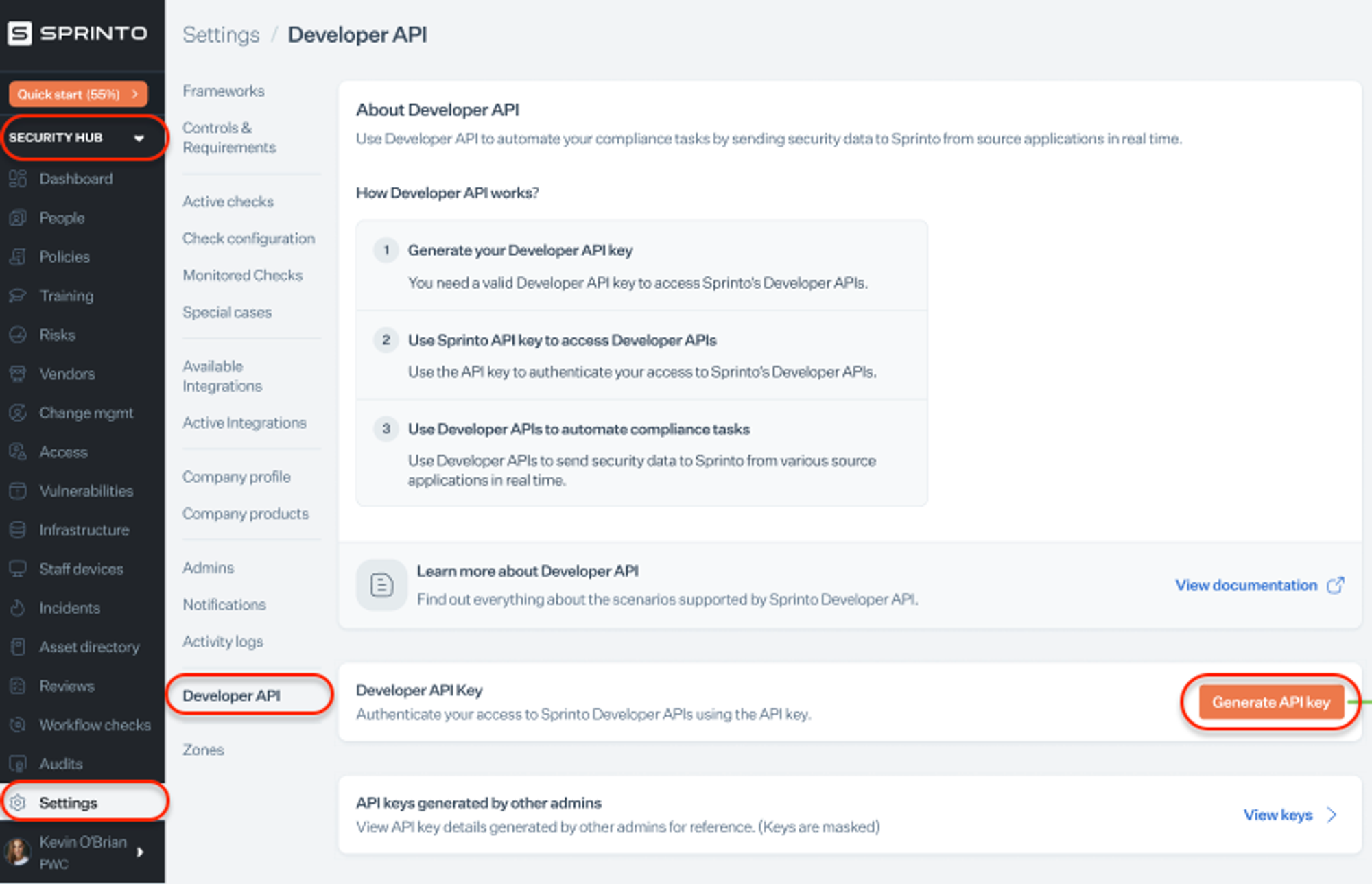
For detailed instructions, refer to our guide on how to generate an API key.
Currently, only the Sprinto admin users can generate the API keys, and all the API keys have complete access to the available graph. As we roll out more features to the Developer API, we'll support limited scopes for each key in the future.
2. Explore the API
Introspection is one of many benefits of using a GraphQL-based API. Introspection allows you to ask the API to describe itself, and the API responds by describing all the available endpoints for calling queries and the type of data you can expect in responses. Ain’t that neat!
But wait, we've got something extra!
We want you to experience and play around with our API in your own comfort zone. Check out our Sprinto API Playground to explore the API directly from your device web browser. Nothing to download, nothing to install.
We want you to experience and play around with our API in your own comfort zone. Check out our Sprinto API Playground to explore the API directly from your device web browser. Nothing to download, nothing to install.
Use the below link based on your current geographic location to explore Sprinto API:
- United States: Sprinto API Playground
- Europe: Sprinto API Playground
- India: Sprinto API Playground
Recommended reading
In case you need help to set up the API Playground, we have got it covered for you.
To make most of the API Playground, we recommend referring to the API structure to understand the valid query and mutation calls, and to the Pagination to understand how to fetch and process paginated records from Sprinto Developer API.
Once you have familiarized yourself with the API and feel comfortable, let's get hands-on!
3. Begin using the API
Writing raw GraphQL queries takes a significant amount of work and time. We recommend using your desired client library to reach the same goal with a way simpler route. Here is the list of the most popular client libraries:
Once you pick your favorite programming language and client, you can set your client like so:
curl 'https://app.sprinto.com/dev-api/graphql' \
-H 'api-key: <your-api-key>' \
-H 'content-type: application/json' \
--data-raw '{"query":"query ExampleQuery {\n hello\n}\n","variables":{},"operationName":"ExampleQuery"}' \
--compressed
pip install requests
import requests
url = 'https://app.sprinto.com/dev-api/graphql'
api_key = '<your-api-key>'
headers = {
'api-key': api_key,
'content-type': 'application/json'
}
data = {
'query': 'query ExampleQuery {\n hello\n}\n',
'variables': {},
'operationName': 'ExampleQuery'
}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 200:
print("Response:")
print(response.json())
else:
print(f"Request failed with status code {response.status_code}")
print(response.text)
npm install axios
const axios = require('axios');
const url = 'https://app.sprinto.com/dev-api/graphql';
const apiKey = '<your-api-key>';
const headers = {
'api-key': apiKey,
'content-type': 'application/json',
};
const data = {
query: 'query ExampleQuery {\n hello\n}\n',
variables: {},
operationName: 'ExampleQuery',
};
axios.post(url, data, { headers })
.then(response => {
console.log('Response:', response.data);
})
.catch(error => {
console.error('Request failed with status code', error.response.status);
console.error(error.response.data);
});
You will notice that all clients require a couple of important arguments: a base URL an authorization mechanism.
-
The base URL for all Sprinto GraphQL API calls is:
Base URL:https://app.sprinto.com/dev-api/graphql
-
In this case, the authorization mechanism is the API key you have generated from the Sprinto app.
Please note again that it is best practice not to inject your API key directly here. This can lead to exposing your API key. The best practice is to make it available to the program via an environment variable or another recommended method of handling secrets. -
Once you are ready, you can make a simple call with your GraphQL payload looking like this:
query ExampleQuery { hello }
-
If you get “Hello world” as a response to the above query, you are set and ready to go!
Updated 12 months ago